When encountering the “invalid decimal literal” error in Python, it is crucial to understand the root cause and how to resolve it effectively. This error typically arises when Python identifiers start with a number, which is not allowed. Let’s delve into this issue and explore the solutions to rectify it.
What Causes the “Invalid Decimal Literal” Error?
The “invalid decimal literal” error occurs due to Python’s syntax rules, specifically regarding identifiers that cannot begin with a number. This restriction is essential for maintaining consistency and clarity in Python code. When a number is mistakenly placed at the beginning of an identifier, Python interprets it as an invalid decimal literal, triggering an error.
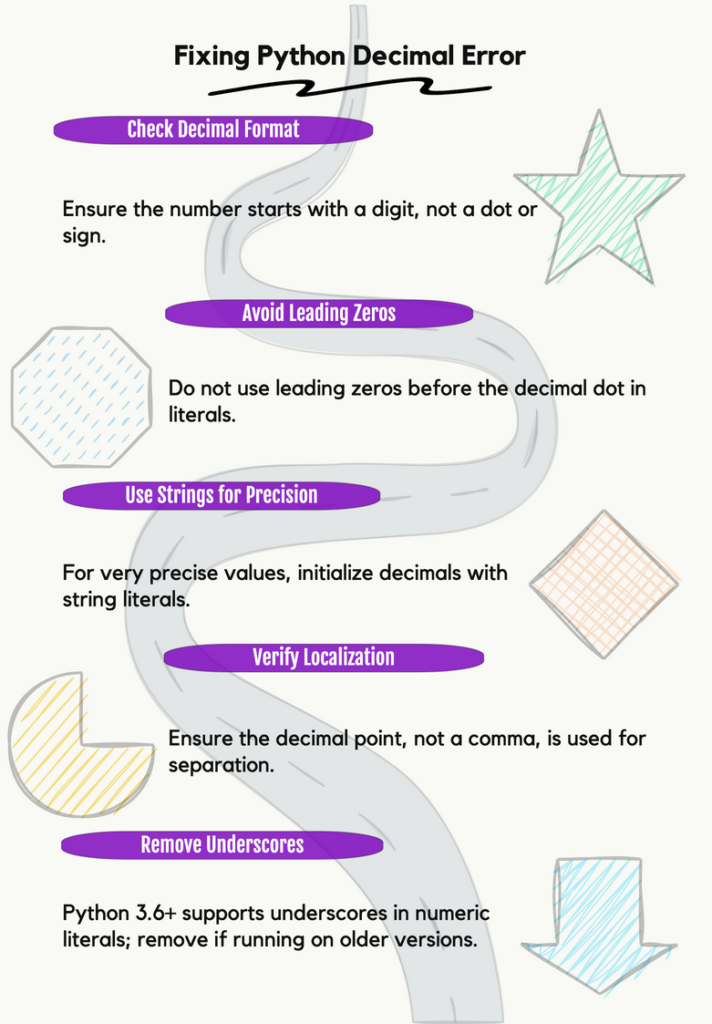
Understanding Python Identifiers
In Python, identifiers are names used to identify variables, functions, classes, modules, or other objects. These names must adhere to certain rules:
- They can contain letters (both uppercase and lowercase), digits, and underscores.
- They cannot start with a digit.
- They are case-sensitive.
Resolving the Error with Examples
Let’s consider some examples to illustrate how this error can occur and how to fix it:
Example 1: Incorrect Identifier Starting with a Number
# Incorrect identifier starting with a number
100_year = date.today().year - age + 100
In this case, the identifier 100_year
violates the rule of starting with a number, leading to the “invalid decimal literal” error.
Solution:
To correct this issue, modify the identifier to comply with Python’s naming conventions:
# Corrected identifier
hundred_year = date.today().year - age + 100
Example 2: Handling Decimal Literals Properly
# Incorrect usage of a decimal literal starting with a number
2for = 15
Here, 2for
is an invalid identifier due to starting with a number.
Solution:
To rectify this error, adjust the identifier as follows:
# Corrected identifier
for_2 = 15
Best Practices for Avoiding Invalid Decimal Literals
To prevent encountering the “invalid decimal literal” error in your Python code, follow these best practices:
- Choose Descriptive Identifiers: Use meaningful names for variables that comply with Python’s naming conventions.
- Avoid Starting with Numbers: Ensure that identifiers do not begin with digits to prevent syntax errors.
- Use Underscores: When needed, use underscores to separate words in identifiers instead of starting with numbers.
By adhering to these guidelines, you can write clean and error-free Python code that is easy to read and maintain.