When working with databases in Python, the filter_by
method is a powerful tool for performing simple queries on column names using regular keyword arguments (kwargs). It allows you to retrieve specific records from a database table based on certain conditions. Let’s dive into how you can utilize filter_by
effectively in your Python code.
Basic Usage of filter_by
The filter_by
method is commonly used with SQLAlchemy, a popular SQL toolkit and Object-Relational Mapping (ORM) library for Python. To use filter_by
, you need to have a database connection established and a table defined. Here’s a basic example:
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.orm import sessionmaker
from sqlalchemy.ext.declarative import declarative_base
# Create a connection to the database
engine = create_engine('sqlite:///example.db')
Session = sessionmaker(bind=engine)
session = Session()
# Define a table model
Base = declarative_base()
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True)
name = Column(String)
country = Column(String)
# Create the table in the database
Base.metadata.create_all(engine)
# Perform a simple query using filter_by
result = session.query(User).filter_by(name='Deb').all()
print(result)
In this example, we define a User
table with columns for id
, name
, and country
. We then use filter_by
to retrieve all users with the name ‘Deb’. The filter_by
method takes keyword arguments, where the column names are the keywords and the values are the desired values to match.
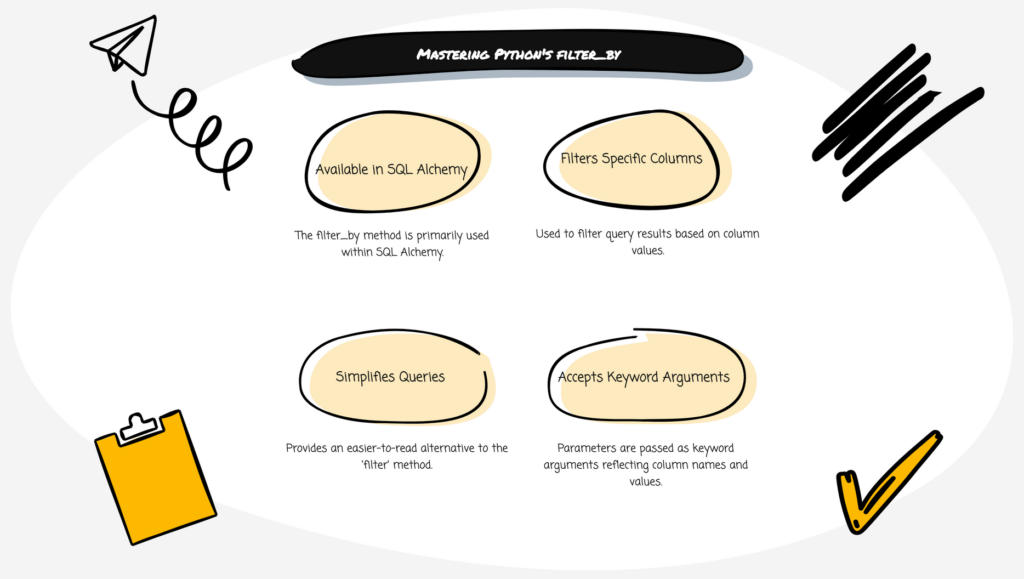
Equivalent Usage with filter
The same query can be accomplished using the filter
method, which allows for more complex expressions. Instead of using kwargs, you can use the ==
equality operator, which has been overloaded on the column objects. Here’s an example:
result = session.query(User).filter(User.name == 'Deb').all()
print(result)
This code snippet achieves the same result as the previous example, but it uses filter
with the ==
operator to compare the name
column with the value ‘Deb’.
Advanced Queries with filter
The filter
method provides more flexibility and power when it comes to constructing complex queries. You can use logical operators like or_
and and_
to combine multiple conditions. Here’s an example:
from sqlalchemy import or_
result = session.query(User).filter(or_(User.name == 'Roy', User.country == 'Canada')).all()
print(result)
In this case, we use the or_
operator to retrieve users whose name is ‘Roy’ or whose country is ‘Canada’. The filter
method allows you to chain multiple conditions together to create more sophisticated queries.
Combining filter_by and filter
You can also combine filter_by
and filter
methods to build even more targeted queries. Here’s an example:
result = session.query(User).filter_by(country='USA').filter(User.name.like('D%')).all()
print(result)
This query retrieves all users from the USA whose names start with the letter ‘D’. We first use filter_by
to select users based on their country, and then we apply an additional filter
condition to match names starting with ‘D’ using the like
operator.